For the latest Dart news, visit our new blog at https://medium.com/dartlang .
We are proud to announce the Milestone 3 release of the Dart SDK, which provides a new, cohesive API for asynchronous programming and a unified model for events. Some of the new or improved classes in this release include Iterable, Stream, and Future.
Dart's M3 release delivers on a common developer request for a more unified approach to events. New and updated in M3, the Iterable class and the Stream class now provide synchronous and asynchronous models, respectively, for a common theme: a sequence of items.
The Iterable class is now much more prominent in Dart and is the ancestor of common collections like List and Set. Many APIs now return Iterable instead of a specific collection. You can map, join, skip, take, and more on an Iterable. Here is an example:
var names = getAccounts().where((a) => a.isPlatinum)
.map((a) => a.fullName)
.toList();
The Stream class (and its dart:async library) is new, and it provides an asynchronous stream of events. An event can be any Dart object, which makes Streams very flexible. Consumers of a Stream can listen for events, and streams can be piped, transformed, filtered, and more. Streams are now the unified way to deliver asynchronous events, and we are working to apply them across HTML, I/O, isolates, and more. Here is an example of using streams with the HTML library, treating clicks as a stream of events:
query('#button').onClick.listen((e) => submitForm());
The Future class, which represents a single value available in the future, was cleaned up and is now much easier to use. In many cases this allows you to write asynchronous code very similar to its synchronous counterpart:
Some of these changes are not backwards compatible. Luckily, you can use Dart Editor's Clean Up feature to help automatically port your code from the old API to the new API. Here is an example of Clean Up at work:
Over the following months we will continue to work on the libraries, applying the new asynchronous models introduced in M3. We always appreciate the feedback, please let us know what you think at dartbug.com and misc@dartlang.org.
To get started, you can download the Dart SDK from dartlang.org, learn about the language and libraries, and follow our tutorials. If you already have Dart Editor, it can auto-update to the latest release for you.
Posted by Florian Loitsch, Software Engineer and Corelib Hacker
Dart's M3 release delivers on a common developer request for a more unified approach to events. New and updated in M3, the Iterable class and the Stream class now provide synchronous and asynchronous models, respectively, for a common theme: a sequence of items.
The Iterable class is now much more prominent in Dart and is the ancestor of common collections like List and Set. Many APIs now return Iterable instead of a specific collection. You can map, join, skip, take, and more on an Iterable. Here is an example:
var names = getAccounts().where((a) => a.isPlatinum)
.map((a) => a.fullName)
.toList();
The Stream class (and its dart:async library) is new, and it provides an asynchronous stream of events. An event can be any Dart object, which makes Streams very flexible. Consumers of a Stream can listen for events, and streams can be piped, transformed, filtered, and more. Streams are now the unified way to deliver asynchronous events, and we are working to apply them across HTML, I/O, isolates, and more. Here is an example of using streams with the HTML library, treating clicks as a stream of events:
query('#button').onClick.listen((e) => submitForm());
The Future class, which represents a single value available in the future, was cleaned up and is now much easier to use. In many cases this allows you to write asynchronous code very similar to its synchronous counterpart:
Synchronous | Asynchronous |
bool writeFile(String data, File file) { try { var io = file.openSync(FileMode.WRITE); io.writeStringSync(data); io.closeSync(); return true; } catch (error) { return false; } } | Future<bool> writeFile(String data, File file) { return file.open(FileMode.WRITE) .then((io) => io.writeString(data)) .then((io) => io.close()) .then((io) => true) .catchError((error) => false); } |
Some of these changes are not backwards compatible. Luckily, you can use Dart Editor's Clean Up feature to help automatically port your code from the old API to the new API. Here is an example of Clean Up at work:
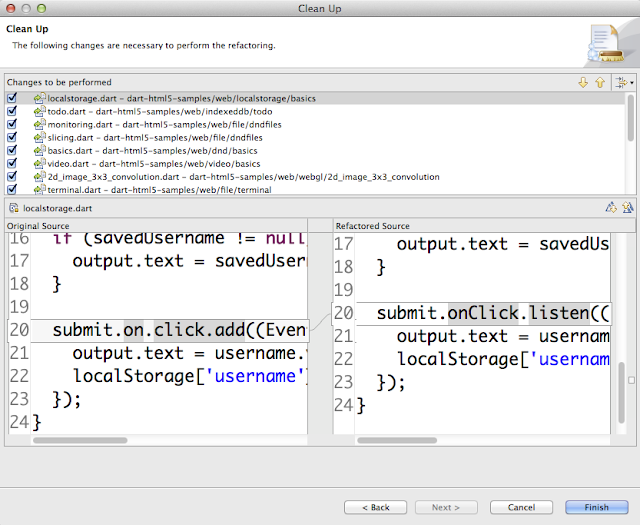
Over the following months we will continue to work on the libraries, applying the new asynchronous models introduced in M3. We always appreciate the feedback, please let us know what you think at dartbug.com and misc@dartlang.org.
To get started, you can download the Dart SDK from dartlang.org, learn about the language and libraries, and follow our tutorials. If you already have Dart Editor, it can auto-update to the latest release for you.
Posted by Florian Loitsch, Software Engineer and Corelib Hacker